CORS – Cross-Origin Resource Sharing – What, how, and why?
Have you ever wondered how API resources can browse safely from domain origins other than the server?
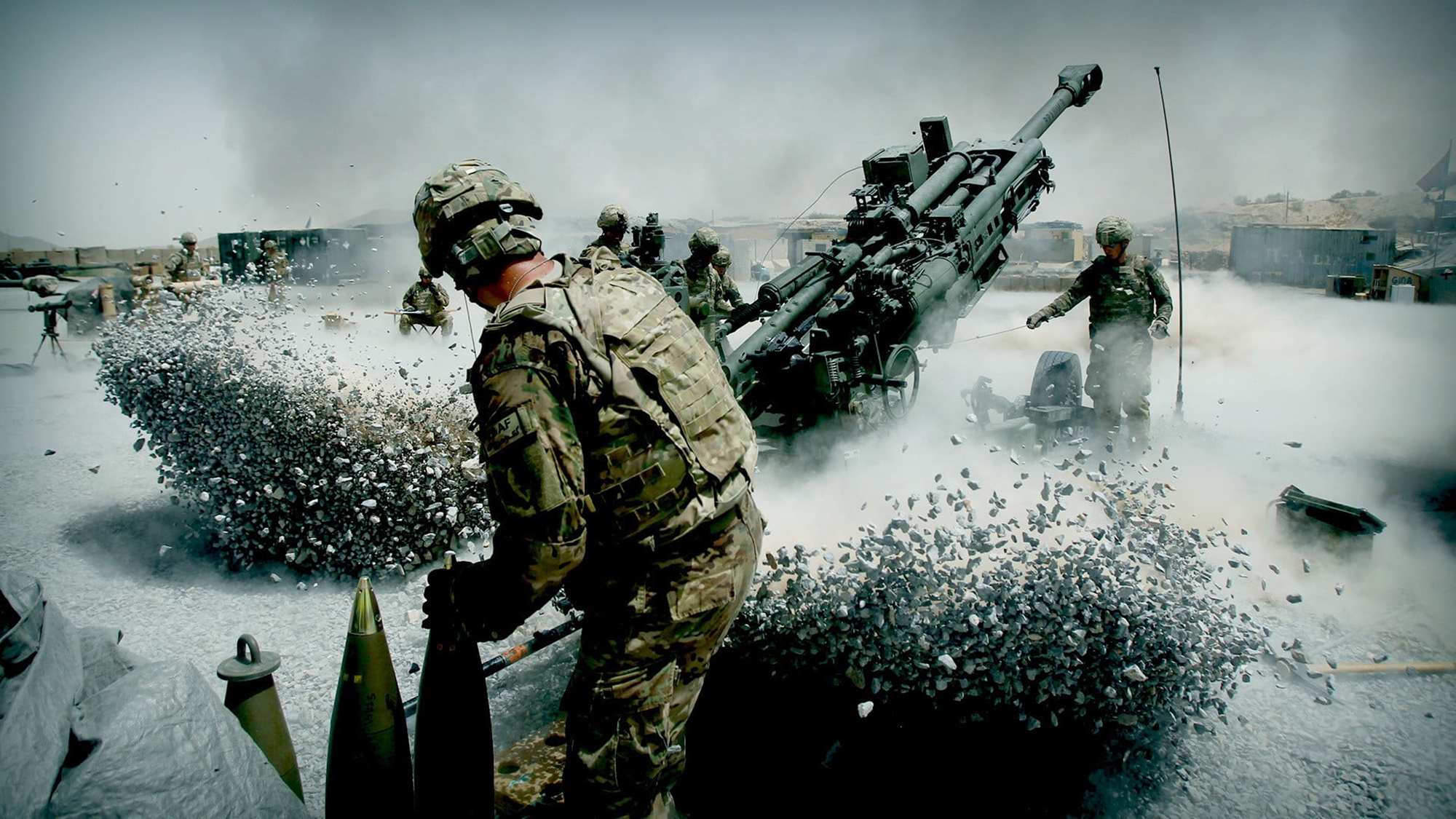
Have you ever wondered how API resources can browse safely from domain origins other than the server? It is possible thanks to Cross-Origin Resource Sharing (CORS), a standard set of HTTP response headers and preflight demands. In addition, CORS enables the usage of specific APIs behind a certain domain.
In this guide, I will try to explain some things that many do not understand, one of which is: "why use CORS?" I have also seen people ask, "what is CORS in web API" in this short read, I would try to answer these questions and discuss other not-so-confusing parts of CORS like "CORS security," "CORS meaning," "CORS headers," and much more.
CORS is not difficult to implement, but it is essential to clearly understand how it works and when it should be used to enable you to implement it correctly in your API. If you want to build an API that can be shared across multiple domains, continue reading this article.
What is CORS?
CORS stands for Cross-Origin Resource Sharing, a system or software framework that allows limited resources on a web page from other domains to be requested as if they were on the same domain server.
CORS is a standard system that supports using APIs behind a certain domain from other sources. CORS has also been described as a mechanism that allows cookies to be sent to domains other than the one to which the page was originally issued. You might have come across CORS in your journey as a developer if you've tried to make an API request that fails with a message like "Access denied," "Bad request," "Origin error," etc. This is because CORS is enabled by default on any new API you create, and the API gateway CORS issues standards on how external client l servers can request access to data on an origin server.
You should understand that CORS is not a sort of technology. It simply is a set of commands that operate on a server to regulate requests and access from external user domains, mostly those of alien origin. It is a way servers and browsers dictate what hosts are allowed access to their contained data and set the conditions under which resources can be accessed. I believe this answers the question:" what is CORS in web API?"
What use does CORS serve?
CORS allows limited resources on a web page from other domains to be requested as if they were on the same domain. CORS is used for Cross-origin requests, that is, if you want to request a resource from a different origin than the one your page is running on.
A page can be running on any one of these four origins:
· Scheme: The protocol used (Example: https, HTTP)
· Host: The domain or server name (Example: example.com, example-server.com)
· Port: The port number (Example: 80, 8080, 443)
· User info: The user identification for the page (Example: username, userid)
CORS confirms that the cross-origin resource data server host would permit the request. Cross-domain traffic increases daily, and accessing data on an alien domain requires an effective API gateway CORS.
In case you still don't know why to use CORS: CORS is useful because it allows you to access an API hosted on one domain on a different domain. It is used mostly to build cross-origin applications (multi-domain applications), where you want to share code between multiple domains. CORS is used to access content on a distant server or domain, i.e., a server other than the server where your current website is located, while keeping content safe on both sides as cross-origin traffic occurs.
How does CORS work?
For example, say we have a website running on www.example.com that is built using React, and we have an API hosted on api.example.com that runs on Express. CORS allows us to use the API running on React, even though the API is hosted on a different server (api.example.com). CORS allows clients on alien domains to send HTTP requests demanding access to data on a domain running on a different server or program.
CORS works by setting up a preflight request, followed by a normal request. Next, CORS lets a user agent (browser/server) request a different server for cross-domain reach to resources stored on it.
A request like XMLHttpRequest can be sent in this case.
Basically, at the point when a program sends out an HTTP request to a server, it forwards an HTTP request header with the certifications of the domain from where it received the URL to the host. If the host is programmed to give cross-domain resource access to that client, it responds with a set of response headers that permits the client access to read the hosted data. The client gets two sorts of response headers from the host:
One is the Access-Control-Allow-Origin (ACAO) header. This header is obligated to the implementation of CORS on the data host. For example, if the host server responds with "Access-Control-Allow-Origin: *," it means the server is programmed to allow access to the resources hosted on it to every domain. This means there is no restriction on who can access this data.
The other header is the Access-Control-Allow-Header header. This one is responsible for restricting servers, domains, or browsers seeking requests; it is sent by hosts restricted to specific domains or URL credentials. The Access-Control-Allow-Header can also come with a programmed list of domains that dictate the domains and users that can view the requested data on the host server or with a command similar to Access-Control-Allow-Origin: https://foo.example, sporting a domain or URL that is permitted to host data.
These CORS headers and measures are implemented to settle CORS security issues and ensure safe cross-domain data sharing across platforms.
CORS requests
A user can make two types of requests to a host protected by CORS - simple requests and preflight requests.
1. Simple requests
work, as the name depicts, when requesting data from a host. All you have to do is specify the server's URL and the method you want to be executed. In this case, the HTTP GET request header is used, as in most cases, to request the data. This way, you either get access to the requested domain or an error message showing that the CORS security denies access to your host domain. And as we mentioned earlier, the API gateway CORS issues access to visitors, so your domain has to be on the Access-Control-Allow-Origin list to be let in, like the RSVP list of an exclusive event.
This, for example, is what a client might send to request data from a server.
const XHR = new XMLHttpRequest();
const url = 'https://bar.other/resources/public-data/';
xhr.open('GET', url);
xhr.onreadystatechange = someHandler;
xhr.send();
OR
GET /resources/public-data/ HTTP/1.1
Host: bar.other
User-Agent: Mozilla/5.0 (Macintosh; Intel Mac OS X 10.14; rv:71.0) Gecko/20100101 Firefox/71.0
Accept: text/HTML,application/xhtml+xml,application/XML;q=0.9,*/*;q=0.8
Accept-Language: en-us,en;q=0.5
Accept-Encoding: gzip,deflate
Connection: keep-alive
Origin: https://foo.example
2. Preflight requests
check the server that houses the needed resource to see if the browser or client-server is allowed access to data stored on that server's origin domain. This means that preflight requests precede the request for data, like checking a ceremony's guest list online before you go there uninvited.
First, we should go through the course of how preflight requests function – when a domain or a site destination is typed into a browser, it checks the domain origin's host server to see if it is allowed access to data resources on that server or domain. It does this by sending an HTTP GET request. The host server checks the request and sends back the data if it is programmed to allow Cross-Origin Resource Sharing with the requesting server, or it responds with a status code: "Method Not Allowed" if Cross-Origin Resource Sharing is not allowed between the servers.
This HTTP GET request can be used in cases of a preflight request:
const XHR = new XMLHttpRequest();
xhr.open('POST', 'https://bar.other/resources/post-here/');
xhr.setRequestHeader('X-PINGOTHER', 'pingpong');
xhr.setRequestHeader('Content-Type', 'text/xml');
xhr.onreadystatechange = handler;
xhr.send('<person><name>Arun</name></person>');
CORS Headers: HTTP Request and Response Headers
1. HTTP request headers:
These request headers are sent by the requesting server/browser (the client) to the host server; the requests commonly use HTTP headers. When a client browser sends out an access request, it is sent via an HTTP request header that contains information about the request (method, URL, etc.), the client's domain, the client's identity, and the client's expectation.
CORS requests contain special headers. They portray the request's origin server and the request type, letting the host server properly evaluate every prerequisite the requests meet before access is allowed.
2. HTTP response headers:
The special HTTP headers that follow CORS responses contain a report about the host server's decision to the access request.
A server response can look something like this:
HTTP/1.1 200 OK
Date: Mon, 01 Dec 2008 00:23:53 GMT
Server: Apache/2
Access-Control-Allow-Origin: *
Keep-Alive: timeout=2, max=100
Connection: Keep-Alive
Transfer-Encoding: chunked
Content-Type: application/XML
[…XML Data…]
CORS construction issues and the rise of CORS vulnerabilities
Some issues will likely occur when CORS is not set up properly on a server. Some of them are CORS security issues. CORS configuration is very critical on your server. If it's not done properly, it may allow malicious user access. Some security issues can arise if CORS is not properly implemented on a server, some of these issues are denial of service attacks, data breaches, resource leaks, buffer overflow, etc. CORS is known to cause issues in various libraries and frameworks due to clumsy setups. It is recommended that a server's CORS and any other cyber security framework be configured by a professional.
A few known CORS vulnerabilities have been detected in the past.
1. CORS Denial of Service (DoS):
Once a malicious user can gain access to your CORS server, then that single access can be manipulated as they can manipulate your server and gain access with a different "origin header," making passage for their DoS botnet.
2. CORS Referrer Leak:
If your CORS-protected server is not properly set up against a referrer leak, then a malicious user can send you their information, which includes their referrer data. CORS Improper Authenticity Protection - If a malicious user sends a request to your server with a valid Origin but an invalid "Host" and "Port" header, then they can request your server.
3. CORS HTTP Response Splitting:
If your CORS security is open to HTTP response splitting, then a malicious user who can request your server with a valid "Origin" header can send you a request with a "Content-Type" header that is different from the one you sent them.
These vulnerabilities, however, can be managed by configuring your API gateway CORS correctly, thereby avoiding all these CORS security issues.
How to prevent CORS-based attacks
There are multiple ways to prevent CORS-based attacks:
· Set up CORS correctly:
This is the most important thing you should do when setting up CORS on your server. Ensure your CORS is set up carefully and by professionals.
· CORS Implementation Best Practices:
You can use the CORS implementation best practices to prevent CORS-based attacks. The best practices ensure that your API is open to access requests while ensuring that the data is properly protected and access is limited to only certain domain origins. It also ensures that preflight requests are properly configured and that your server is well protected against cross-site/cross-domain forgery.
· CORS Proxy:
You can use a CORS proxy to protect your server from CORS-based attacks. CORS proxy allows your traffic to escape errors and attacks between request and response that might want to rig a request forgery by hijacking valid domain traffic.
How to enable CORS
· One can enable CORS either on the client or server sides.
· Enable CORS on the server side
· If you host your API on a server, you can enable CORS on the server side. Enable CORS on the client side
· If you are building a client app, you should enable CORS on the client side.
Conclusion
The internet as we know it is occupied by many, the regular users, the builders, and the perpetrators alike. Various server managers have various measures they implement to keep their servers protected and free from harm, and CORS security is just one of the many measures. It allows them to police the traffic on their servers while keeping their private data private.
Of course, CORS can be attacked too, but as with every cyber security measure, there's always a way to prevent such attacks. This read must have shown you ways of CORS prevention.
CORS is useful because it allows you to use one API hosted on one domain on a different domain. CORS is used mostly to build multi-domain applications, where you want to share code between multiple domains. Some issues may occur when you set up CORS on your server. You can prevent CORS-based attacks by setting up CORS correctly or using a CORS proxy. You can enable CORS either on the server side or the client side.